Matthew Riley
mgriley97@gmail.com
Vulkan Tree Generator
Overview
As my final project for a procedural graphics class, I made a simulation of tree growth. Starting with a flat mesh and a “seed” point, the program iteratively transforms the points to sculpt a simple tree.
I used OpenGL for the first version then rewrote the project in Vulkan after the class ended. I’ll show some demos then describe how it works.
Links:
Demos
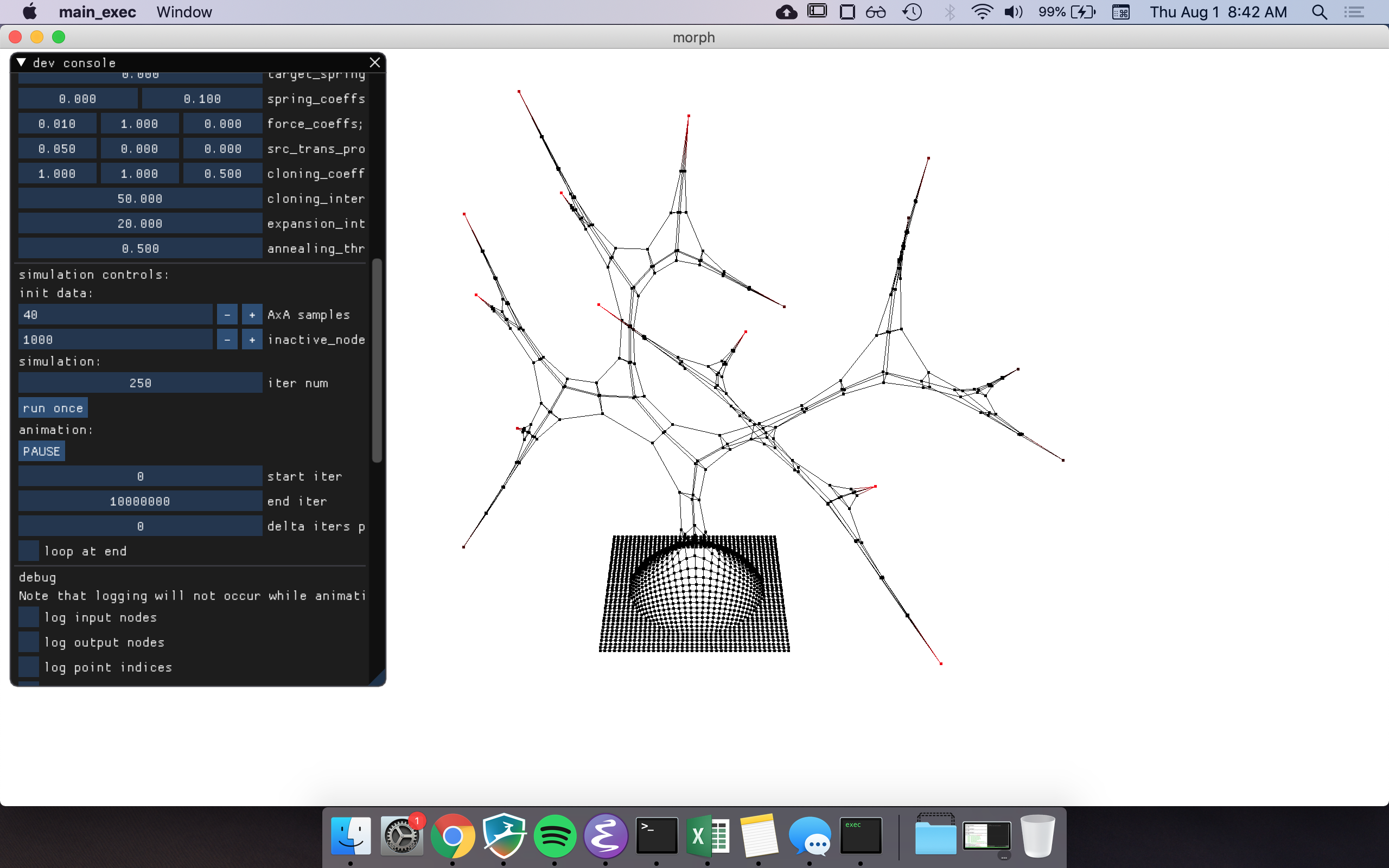
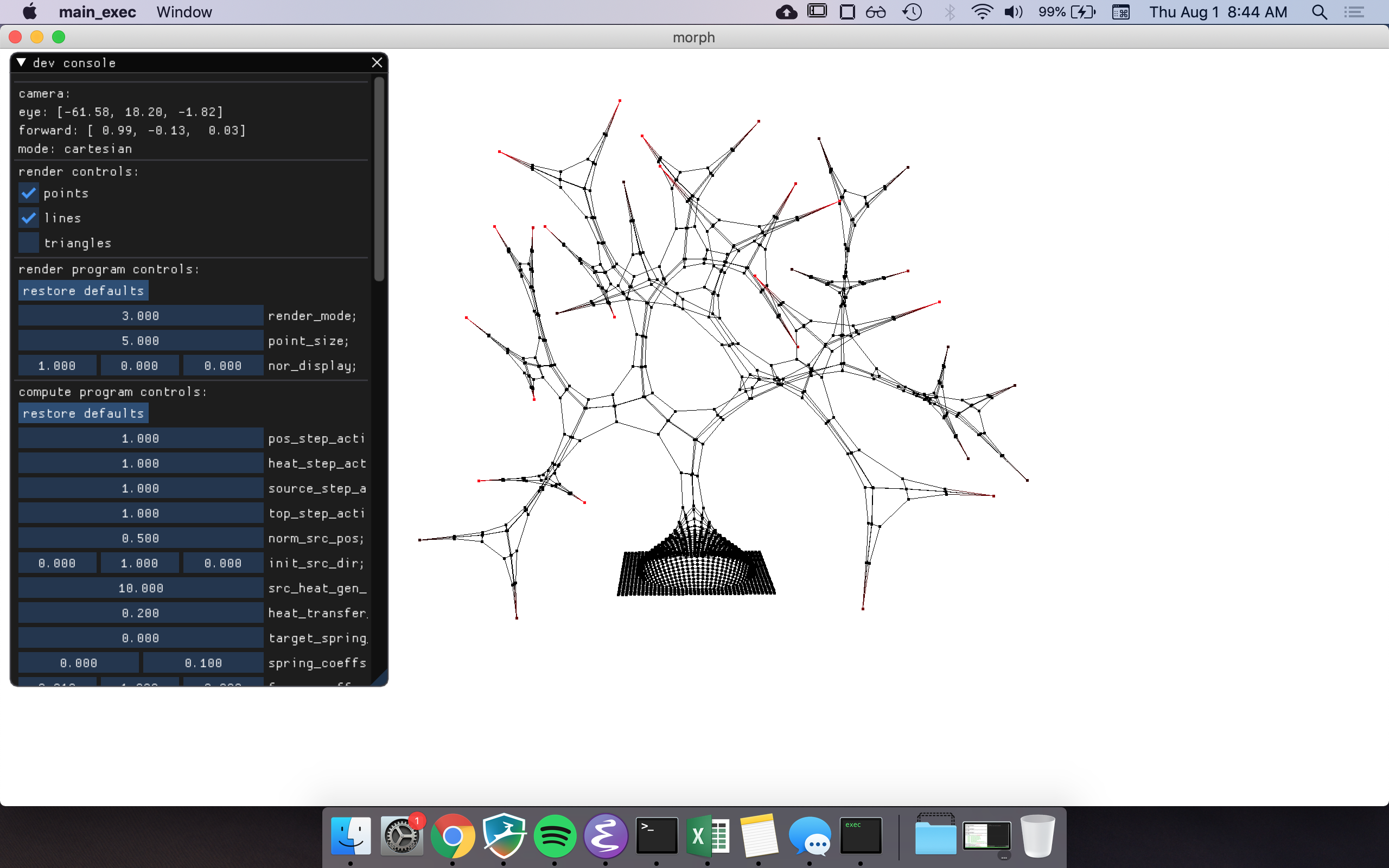
Strategy
My goal was to create a tree-like form. In the graphics class, we learned to do this using L-systems to piece together cylindrical branches. I wanted to try a different way that better represented actual tree growth, to see if I could get more organic, chaotic tree forms.
You start with a flat mesh with a “seed” node in the middle. The seed grows up, pulling/shaping the mesh along with it, then splits off into two seed nodes, each growing in a different direction. Repeat for many iterations to get multiple branches. The mesh anneals over time when there are no seed nodes nearby, giving a sturdy trunk. Nodes are dynamically added to the mesh around the seeds so that they can grow out properly.
The Code
There are three steps to the simulation:
- Generate initial data on the CPU and write it to one of two buffers on the GPU.
- Second, we run a compute shader for
n
iterations. Each iteration, every node updates in parallel. The compute shader is essentially a step function that given a node’s current data (read from Buffer A) computes the node’s next data and writes it to Buffer B. We swap buffers for the next iteration. Between iterations, the CPU does not need to read any data from the GPU. - Third, once all iterations are done, we transfer the buffer data from the GPU to the CPU and render.
Regrettably, some heretical things had to be done in the computer shade to be able to splice new nodes into the tree mesh during an iteration. If you’re interested in the details, please see the code on Github.
In hindsight, I got a bit carried away trying to do this all on the GPU, but I did learn some Vulkan. It would have been simpler to run the tree growth simulation on the CPU and simply write all the node data to a file to play it back later. I think the technique has potential and I’ll probably come back to it at some point. I’m confident that, with some improvements, it’s possible to get much better results than the crude trees shown here.
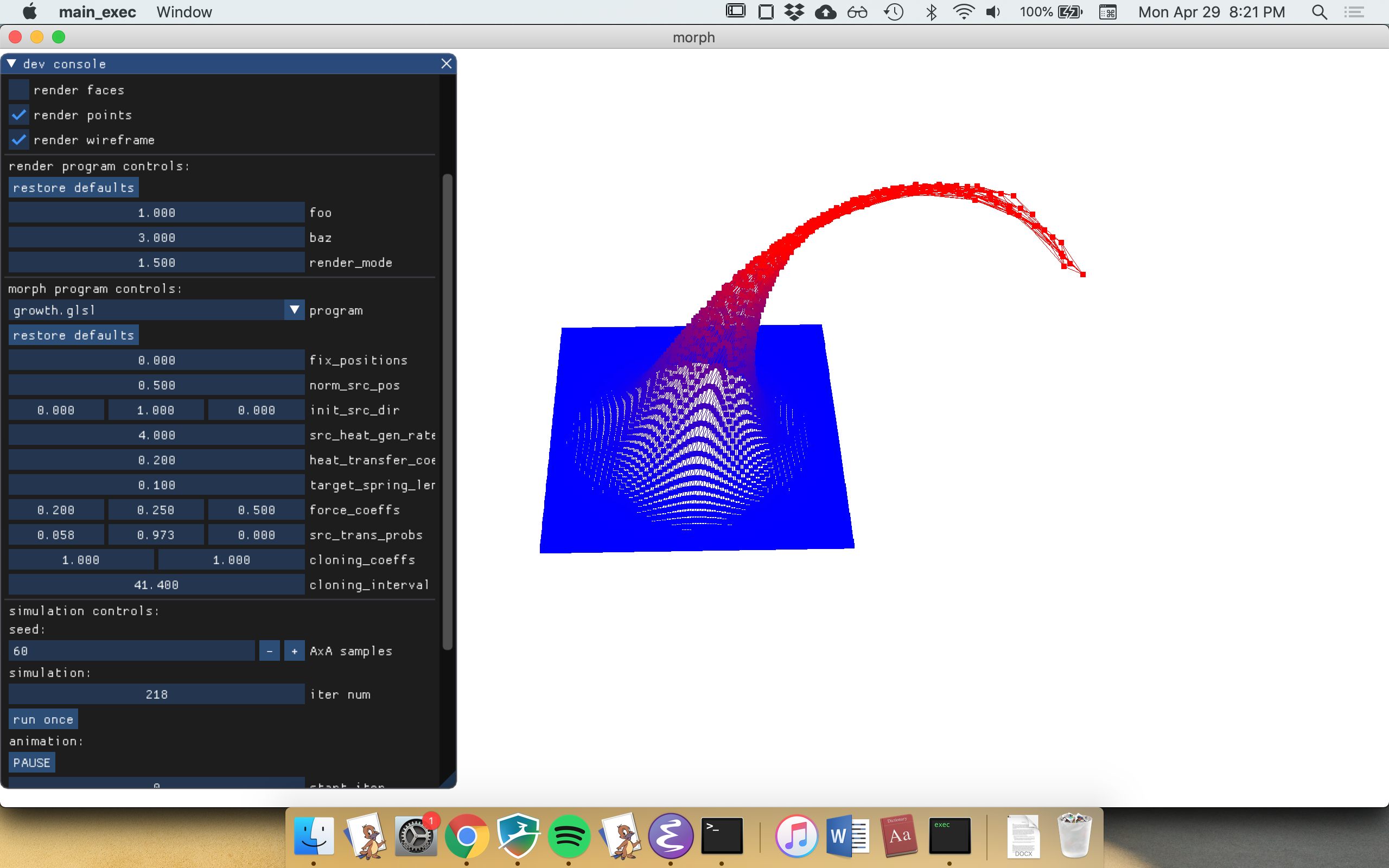
Regions of high heat in red and low heat in blue.
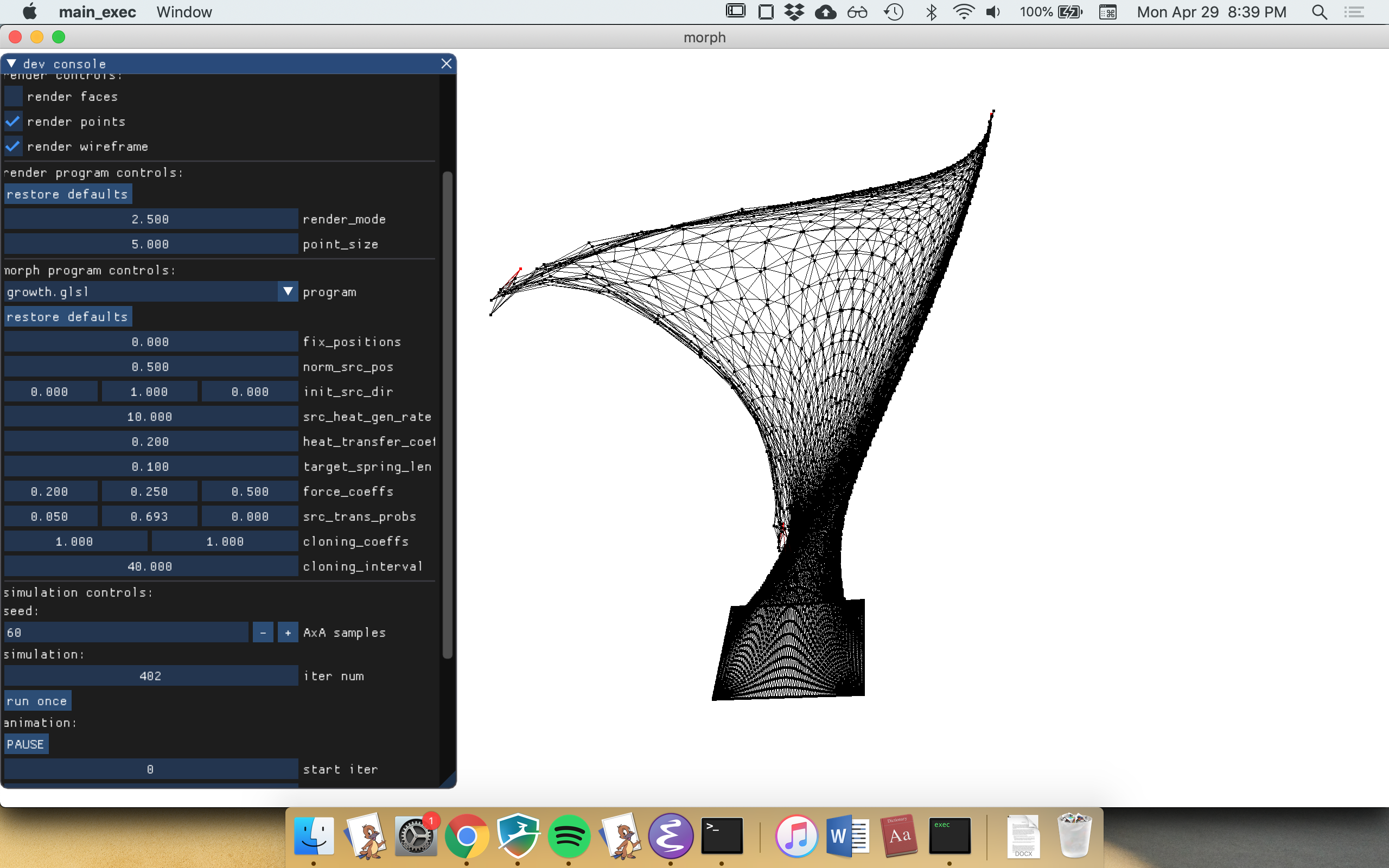
An earlier version of the simulation, before implementing annealing.
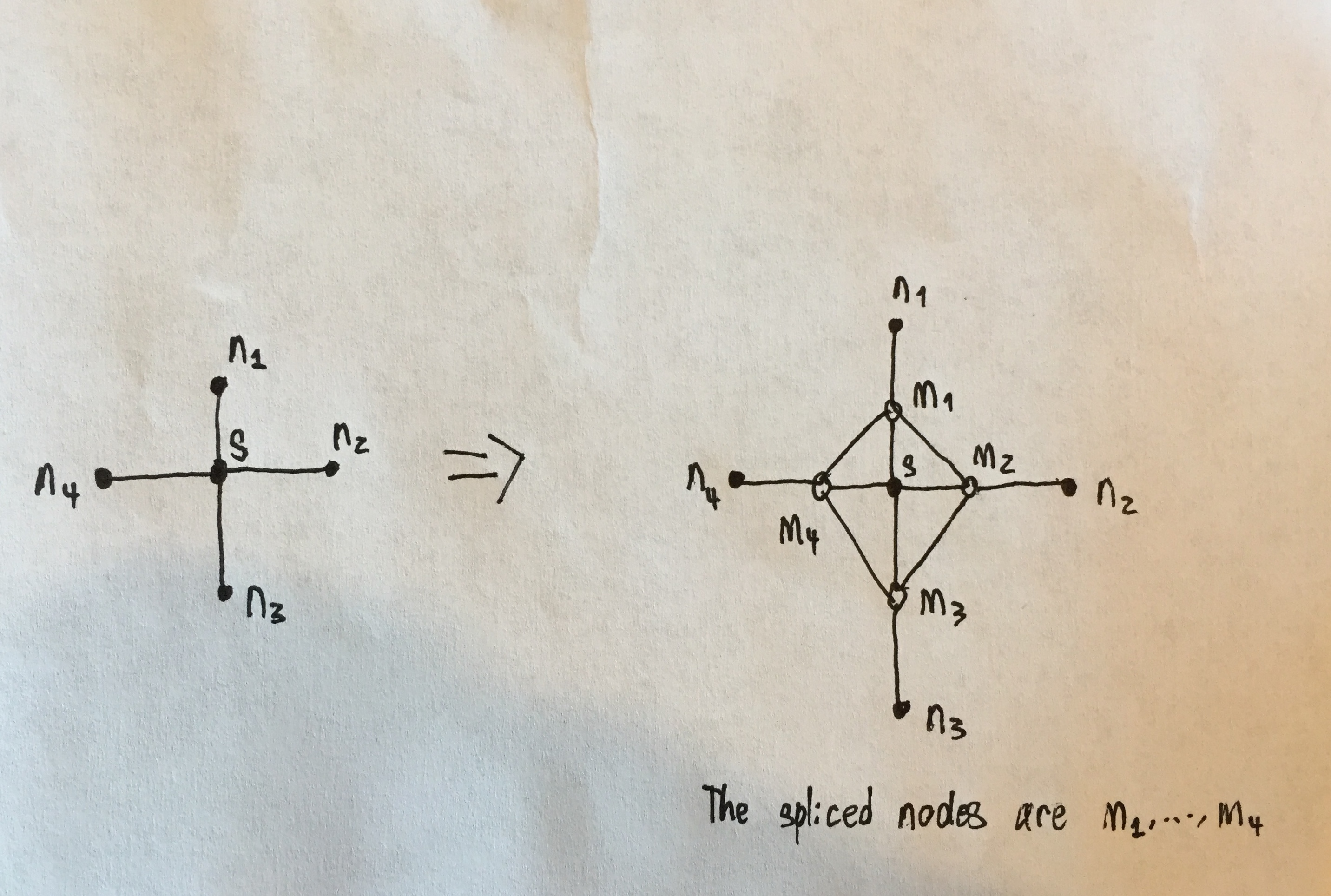
Expansion about a source node